Android Development Tutorial
Customized Progress Bar
In Android we can customize the Progress Bar and make it more attractive and beutifull.
Customize Progress Bar Example
Here I have used same example of file downloading explained in my previous post Android Progress Bar Example , But in this post I have customized the Progress Bar.
How to Customize Progress Bar
To customize a progress bar we need to define the properties of progress bar like Color, Width, Start Color, End Color, Angle etc.These properties are defined in .xml file inside drawable folder.
1: Create a new Android Project
2: Create a folder drawable inside res folder
3: Create custom_progressbar.xml file in drawable (If rawable folder is not there create a drawable folder inside res Folder) folder and set it as progress drawable for Progress Bar (See the Highlighted line in Code in ProgressBarActivity.java)
See the difference in Normal and Customized Progress Bar
Normal/Default Progress Bar Customized Progress Bar
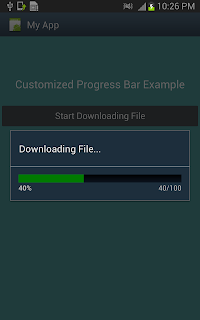
custom_progressbar.xml
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<!-- Define the background properties like color etc -->
<item android:id="@android:id/background">
<shape>
<gradient
android:startColor="#000001"
android:centerColor="#0b131e"
android:centerY="1.0"
android:endColor="#0d1522"
android:angle="270"
/>
</shape>
</item>
<!-- Define the progress properties like start color, end color etc -->
<item android:id="@android:id/progress">
<clip>
<shape>
<gradient
android:startColor="#007A00"
android:centerColor="#007A00"
android:centerY="1.0"
android:endColor="#06101d"
android:angle="270"
/>
</shape>
</clip>
</item>
</layer-list>
main.xml
<LinearLayout
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical"
android:background="#4D9494"
xmlns:android="http://schemas.android.com/apk/res/android">
<TextView
android:id="@+id/textView1"
android:layout_marginTop="80dp"
android:gravity="center_horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Customized Progress Bar Example"
android:textAppearance="?android:attr/textAppearanceLarge" />
<Button
android:id="@+id/button1"
android:layout_marginTop="25dp"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Start Downloading File"
android:onClick="startProgressDialog"/>
</LinearLayout>
ProgressBarActivity.java
public class MainActivity extends Activity
{
ProgressDialog progressBar;
private int progressBarStatus = 0;
private Handler progressBarHandler = new Handler();
private long fileSize = 0;
@Override
public void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
public void startProgressDialog(View V)
{
// prepare for a progress bar dialog
progressBar = new ProgressDialog(V.getContext());
progressBar.setCancelable(true);
progressBar.setMessage("Downloading File...");
progressBar.setProgressStyle(ProgressDialog.STYLE_HORIZONTAL);
progressBar.setProgress(0);
progressBar.setMax(100);
// Get the Drawable custom_progressbar
Drawable customDrawable= res.getDrawable(R.drawable.custom_progressbar);
// set the drawable as progress drawavle
progressBar.setProgressDrawable(customDrawable);
progressBar.show();
//reset progress bar status
progressBarStatus = 0;
//reset filesize
fileSize = 0;
new Thread(new Runnable() {
public void run() {
while (progressBarStatus < 100) {
// process some tasks
progressBarStatus = fileDownloadStatus();
// sleep 1 second to show the progress
try {
Thread.sleep(1000);
}
catch (InterruptedException e) {
e.printStackTrace();
}
// Update the progress bar
progressBarHandler.post(new Runnable() {
public void run() {
progressBar.setProgress(progressBarStatus);
}
});
}
// when, file is downloaded 100%,
if (progressBarStatus >= 100) {
// sleep for 2 seconds, so that you can see the 100% of file download
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
// close the progress bar dialog
progressBar.dismiss();
}
}
}).start();
}
//method returns the % of file downloaded
public int fileDownloadStatus()
{
while (fileSize <= 1000000) {
fileSize++;
if (fileSize == 100000) {
return 10;
} else if (fileSize == 200000) {
return 20;
} else if (fileSize == 300000) {
return 30;
}
else if (fileSize == 400000) {
return 40;
} else if (fileSize == 500000) {
return 50;
} else if (fileSize == 600000) {
return 60;
}
// write your code here
}
return 100;
}
}
Advance Android Topics
Customizing Toast In Android
Showing Toast for Longer Time
Customizing the Display Time of Toast
Using TimePickerDialog and DatePickerDialog In android
Animating A Button In Android
Populating ListView With DataBase
Customizing Checkboxes In Android
Increasin Size of Checkboxes
Android ProgressBar
Designing For Different Screen Sizes
Handling Keyboard Events
More Android Topics:
Android : Introduction
Eclipse Setup for Android Development
Configuring Eclipse for Android DevelopmentBegging With Android
Creating Your First Android ProjectUnderstanding Android Manifest File of your android app
Working With Layouts
Understanding Layouts in AndroidWorking with Linear Layout (With Example)
Nested Linear Layout (With Example)
Table Layout
Frame Layout(With Example)
Absolute Layout
Grid Layout
Activity
Activity In AndroidActivity Life Cycle
Starting Activity For Result
Sending Data from One Activity to Other in Android
Returning Result from Activity
Working With Views
Using Buttons and EditText in AndroidUsing CheckBoxes in Android
Using AutoCompleteTextView in Android
Grid View
Toast
Customizing Toast In AndroidCustomizing the Display Time of Toast
Customizing Toast At Runtime
Adding Image in Toast
Showing Toast for Longer Time
Dialogs In Android
Working With Alert DialogAdding Radio Buttons In Dialog
Adding Check Boxes In Dialog
Creating Customized Dialogs in Android
Adding EditText in Dialog
Creating Dialog To Collect User Input
DatePicker and TimePickerDialog
Using TimePickerDialog and DatePickerDialog In androidWorking With SMS
How to Send SMS in AndroidHow To Receive SMS
Accessing Inbox In Android
ListView:
Populating ListView With DataBaseMenus In Android
Creating Option MenuCreating Context Menu In Android
TelephonyManager
Using Telephony Manager In AndroidWorking With Incoming Calls
How To Handle Incoming Calls in AndroidHow to Forward an Incoming Call In Android
CALL States In Android
Miscellaneous
Notifications In AndroidHow To Vibrate The Android Phone
Sending Email In Android
Opening a webpage In Browser
How to Access PhoneBook In Android
Prompt User Input with an AlertDialog
Storage: Storing Data In Android
Shared Prefferences In Android
SharedPreferences In AndroidFiles: File Handling In Android
Reading and Writing files to Internal StoarageReading and Writing files to SD Card
DataBase : Working With Database
Working With Database in AndroidCreating Table In Android
Inserting, Deleting and Updating Records In Table in Android
How to Create DataBase in Android
Accessing Inbox In Android
I require custom android app development services. On the off chance that you have any thought concerning such affiliation that goes on such associations then let me know.
ReplyDeletewhere we use button onclicklistner
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteVery beautifully explained nice blog buddy.Are you among one of those who are searching for the dedicated developers for Flutter web application Development Then you've come to the right place because we have a dedicated development team that is a specialist in this area. So, what are you waiting for? Visit the official website or email us.
ReplyDeleteIncubasys is an app development company specialist in handyman app development, real estate app development and news app development in Australia! Moreover, we also develop car rental apps, construction apps and jewelry apps!
ReplyDeletethis was a very well written article, the information was very nice Thanks for your efforts
ReplyDeletemeal kit delivery business
Thanks for a good share. If you wanna know more about mobile app development and its best services, click here https://bit.ly/2YqK1mN and get utilized.
ReplyDeleteYour blog is very interesting. Your level of thinking is good and the clarity of writing is excellent. I enjoyed reading this post. I am also a blogger, You can visit our services here Android App Development Services
ReplyDeleteYou can also contact here, if you are looking forward to Hire Android App Developers or Mobile App Development Company
Great Blog!
ReplyDeleteThanks for sharing this excellent blog
If you are looking for
Flutter Mobile App Development t then you are at the right place Then you've come to the right place because we have a dedicated development team that is a specialist in this area. So, what are you waiting for? Visit the official website or email us.
Flutter Developer
In this blog post, you have explained step-by-step how to customize the appearance and behavior of a progress bar, including changing colors, adding animations, and handling progress updates. It's a valuable resource for Android developers looking to enhance the visual appeal and user experience of their apps by creating unique and personalized progress bars. Thanks for sharing this great article, You can also contact us here if you are looking forward to hire mobile application developers, and we will be happy to help you.
ReplyDelete